Performance Testing With Jest: Evaluating The Speed And Efficiency Of Your Code
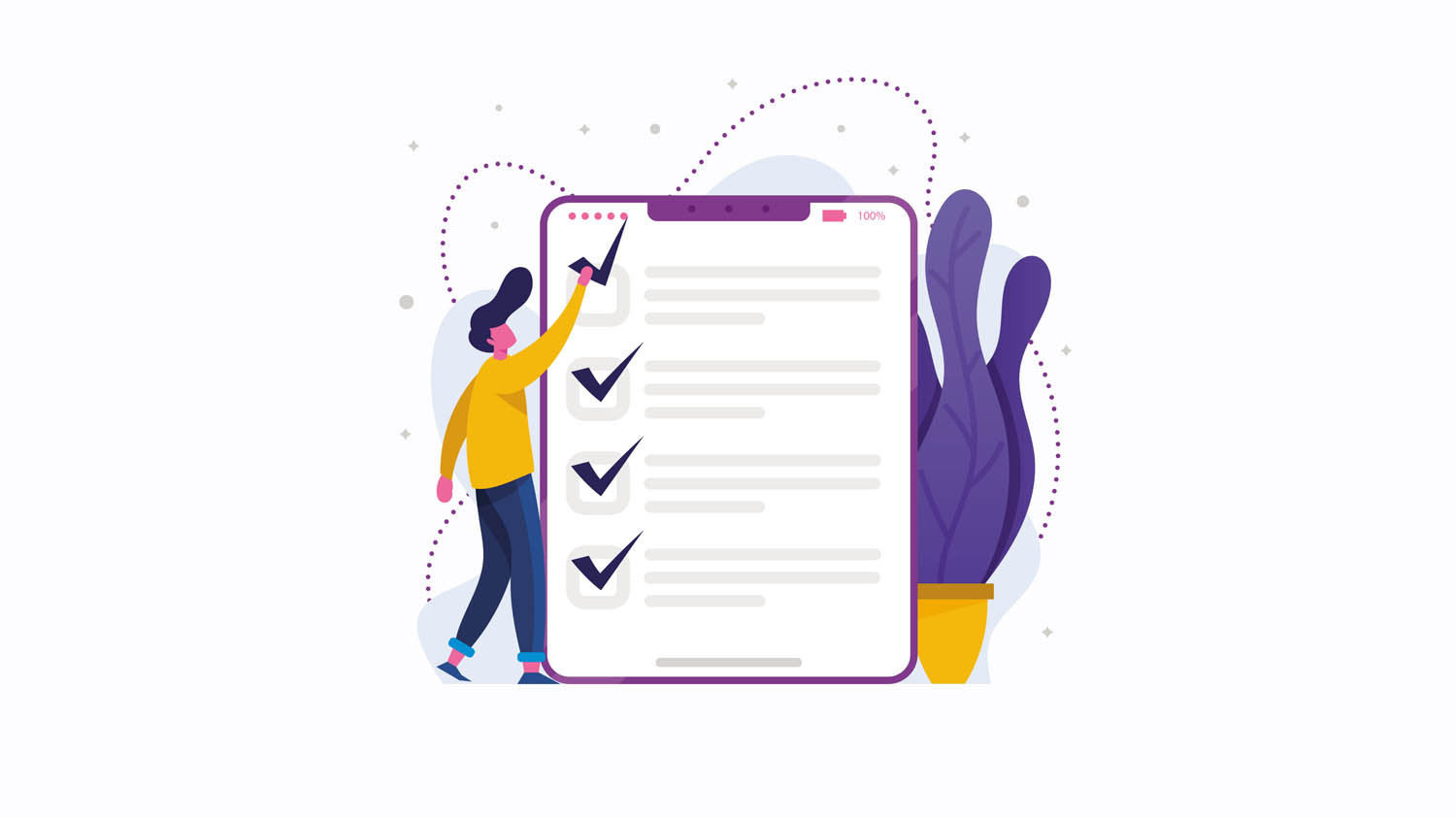
In today’s fast-paced software development world, ensuring your code performs optimally is crucial to delivering a seamless user experience. Performance testing is a critical aspect of software development that helps you evaluate the speed and efficiency of your code. This guide will delve into performance testing using Jest, a popular JavaScript testing framework. Whether you’re a seasoned developer or just starting, this guide will provide you with practical insights, best practices, and techniques to measure and analyze the performance of your code using Jest.
From understanding the fundamentals of performance testing to leveraging Jest’s powerful features, this guide will equip you with the knowledge and tools. These resources can help you identify and fix performance bottlenecks, optimize your code, and ensure your applications run smoothly and efficiently.
Fundamentals of Performance Testing
Software programs or systems are put through performance testing to see if they fulfill predetermined performance benchmarks. Performance testing seeks to locate potential performance bottlenecks, pinpoint problem areas, and guarantee that the system operates at its best under anticipated and unforeseen loads. We’ll examine the principles of performance testing in this comprehensive guide.
- Performance Testing Objectives: The objectives of performance testing are to identify potential bottlenecks, evaluate the performance characteristics of a system or application, establish benchmarks, and optimize performance.
- Types of Performance Testing: There are several types of performance testing, including load testing, stress testing, endurance testing, spike testing, and scalability testing. Each of these tests focuses on different aspects of system performance.
- Load Testing: Load testing involves simulating a user load on a system or application to measure response times and resource utilization. Load testing aims to identify performance bottlenecks and optimize the system or application to handle the expected user load.
- Stress Testing: Stress testing involves pushing a system or application beyond its normal operating limits to measure its behavior under stress. Stress testing aims to identify a system or application’s breaking point and measure how it recovers from failures.
- Endurance Testing: Endurance testing involves running a system or application under a sustained load to measure its performance. Endurance testing aims to identify any performance degradation over time and optimize the system or application to handle sustained loads.
- Spike Testing: Spike testing involves simulating sudden spikes in user load to measure how a system or application responds to sudden increases in traffic. Spike testing aims to identify any performance bottlenecks that occur during sudden spikes in user load.
- Scalability Testing: Scalability testing involves measuring how well a system or application scales as user load increases. Scalability testing aims to identify the maximum number of users a system or application can handle before the performance degrades.
- Performance Metrics: Performance metrics are used to measure the performance of a system or application. The most common performance metrics include response time, throughput, error rate, and resource utilization.
- Test Environment: A test environment replicates the production environment used for performance testing. The test environment should be configured to match the production environment as closely as possible.
Performance testing tools simulate user loads, generate test data, and measure performance metrics. Some popular performance testing tools include Apache JMeter, LoadRunner, and Gatling.
Setting Up Performance Testing with Jest
Setting up performance testing with Jest involves several steps to ensure that you can measure and optimize the performance of your JavaScript code. Here’s a detailed overview of the process:
Install Jest
Jest is a popular JavaScript testing framework that provides built-in support for performance testing. You can install Jest as a development dependency in your project using a package manager like npm or Yarn.
Set up Jest Configuration
Once Jest is installed, you need to configure it for performance testing. You can create a jest.config.js file in your project’s root directory and specify the configuration options for Jest. For example, you can set the testMatch option to specify the pattern for performance test files and the testEnvironment option to set up a suitable environment for performance testing.
Create Performance Test Files
Test files specifically designed to gauge the performance of your code are called performance test files. Using Jest’s test syntax, you can create performance test files that measure performance metrics like execution time, resource usage, and throughput rather than testing for expected results. Performance metrics can be measured in your test files using the performance API integrated into Jest.
Define Performance Benchmarks
You must establish performance benchmarks to gauge the performance of your programs. Benchmarks for performance are the goals you want your code to hit in terms of performance. By establishing allowable thresholds for performance measurements, you can define performance benchmarks. You may, for instance, establish a minimum or maximum acceptable response time criterion.
Run Performance Tests
You can use Jest to execute your performance tests after creating performance test files and specifying performance benchmarks. Your performance tests will be run by Jest, which will also calculate the performance metrics listed in your test files. The measured performance metrics can then be compared to your performance benchmarks to see if your code satisfies the required performance standards.
Analyze Test Results
You can examine the test results following the execution of the performance tests to locate any performance bottlenecks. The performance measurements can be viewed and contrasted with the performance benchmarks using Jest’s built-in reporting facilities. Your code performs well if the measured performance metrics are within the allowable bounds. If you still need to, you should increase the performance of your code.
Optimize Code for Performance
Based on the test results analysis, you may need to optimize your code to address any identified performance bottlenecks. This could involve optimizing algorithms, improving data structures, optimizing database queries, or making other optimizations to your code to improve its performance. You can then rerun the performance tests to verify if the optimizations have improved the performance of your code.
Repeat Testing and Optimization
Iterative processes are used in performance testing. If you want to be sure that your code regularly satisfies the desired performance standards, you might need to repeat the performance testing and optimization procedures several times. Until your code runs at its best, this may entail tweaking your performance benchmarks, applying additional optimizations, and retesting.
LambdaTest is a unified intelligent digital experience testing cloud platform that integrates with Jest, a popular JavaScript testing framework. It enables developers and testers to run their Jest tests over 3000+ browser and operating system combinations. LambdaTest allows developers to identify and fix issues quickly and ensure that their code functions correctly across multiple environments. Lambdatest also enables you to use different test automation tools and frameworks like Selenium, Playwright, etc.
Measuring Performance with Jest
Measuring performance with Jest involves using Jest’s built-in performance API to measure various performance metrics in your performance test files. Here’s a detailed overview of how you can measure performance with Jest:
- Start Performance Measurement: You can use Jest’s performance in your performance test files.start() method to measure performance. This sets a performance mark at the current time, which serves as the starting point for measuring performance metrics.
- Execute Code Under Test: After starting performance measurement, you can execute the code under test, which could be a function, a module, or any other piece of JavaScript code you want to measure for performance. You can use standard JavaScript function calls or import and use modules as needed.
- Stop Performance Measurement: Once the code under test has completed execution, you can use Jest’s performance.stop() method to stop measuring performance. This sets another performance mark at the current point in time, which serves as the ending point for measuring performance metrics.
- Measure Performance Metrics: You can use Jest’s performance after stopping performance measurement.measure() method to calculate various performance metrics based on the starting and ending points. You can specify the name of the performance metric, the starting mark, and the ending mark as arguments to this method.
- Define Performance Benchmarks: In your performance test files, you can define performance benchmarks by setting acceptable thresholds for performance metrics. For example, you can define a threshold for maximum acceptable response time, minimum acceptable throughput, or maximum acceptable resource utilization.
- Compare with Performance Benchmarks: After measuring performance metrics, you can use Jest’s built-in expect API to compare the measured performance metrics against the performance benchmarks. You can use Jest’s matchers, such as toBeLessThan(), toBeGreaterThan(), toBeCloseTo(), etc., to make assertions on the performance metrics.
- Reporting and Analysis: Jest provides built-in reporting features that allow you to view the performance metrics and their comparison against the performance benchmarks. You can use the test results and the performance metrics to analyze the performance of your code and identify potential performance bottlenecks.
Optimizing Code Performance
Optimizing code performance is critical to software development to ensure that your code runs efficiently and meets the desired performance requirements. Here are some general tips and best practices for optimizing code performance:
- Profile Your Code: Profiling is measuring and analyzing the performance of your code to identify performance bottlenecks. Use profiling tools and techniques, such as performance profilers, memory profilers, and code profiling libraries, to gain insights into the performance characteristics of your code. This can help you identify areas that need optimization.
- Algorithm and data structure optimization: Pick effective algorithms and data structures suitable for the particular issue you are attempting to solve. Use data structures that are memory and access time efficient and lower time complexity techniques. This may have a big effect on the overall functionality of your code.
- Optimize Loops and Iterations: Loops and iterations are often a significant source of performance overhead. Optimize loops by reducing unnecessary iterations, avoiding redundant calculations, and using appropriate loop constructs (e.g., for loop instead of forEach or map for large arrays). Also, use efficient iteration techniques, such as loop unrolling or iterators, where applicable.
- Minimize I/O Operations: Input/Output (I/O) operations, such as reading from or writing to files or making network requests, can be expensive in terms of time and resources. Minimize I/O operations by batching requests, using efficient I/O libraries, and optimizing file or network access patterns.
- Optimize Memory Usage: Efficient memory management is crucial for performance optimization. Avoid unnecessary memory allocations, minimize memory leaks, and optimize data storage and retrieval from memory. Use techniques like object pooling, lazy loading, and caching to optimize memory usage.
- Optimize Database Operations: Database operations can significantly impact code performance. Optimize database queries by minimizing the number of queries, optimizing the schema design, and using appropriate indexing. Also, use database caching and caching strategies to reduce the load on the database.
- Optimize CPU Usage: CPU-bound operations, such as complex calculations or heavy computation, can impact code performance. Optimize CPU usage using optimized algorithms, avoiding redundant computations and optimizing parallel processing where applicable.
- Use Asynchronous Programming: Asynchronous programming can improve code performance by allowing concurrent execution of tasks and avoiding blocking operations. Use asynchronous programming techniques, such as callbacks, promises, or async/await, to optimize code performance, especially for I/O-bound operations.
- Optimize Code for the Target Platform: Consider the target platform and its constraints when optimizing code performance. For example, optimize code differently for web applications compared to mobile applications or embedded systems. Consider factors such as hardware resources, operating systems, and platform-specific optimizations.
- Benchmark and Test: Regularly benchmark and test your code to measure its performance and identify any performance regressions. Use performance testing tools and frameworks, such as Jest, benchmark.js, or JUnit, to validate the performance of your code against defined benchmarks and make necessary optimizations.
Best Practices for Performance Testing with Jest
Performance testing with Jest, a popular JavaScript testing framework, can help identify and address performance bottlenecks in your code. Here are some best practices for performance testing with Jest:
- Define Clear Performance Goals: Define your performance goals and expectations before starting performance testing with Jest. This includes defining acceptable response times and resource utilization levels. Clear performance goals will help guide your testing efforts and ensure that you are testing against meaningful criteria.
- Use Realistic Test Data: Use realistic and representative test data in your performance tests. Real-world data can help simulate usage scenarios and provide more accurate performance measurements. Avoid using synthetic or overly simplified data that may not reflect real-world scenarios.
- Reproduce Production-like Environments: Create performance testing environments that resemble your production environment. This includes using similar hardware, software, and network configurations. Reproducing production-like environments ensures that your performance tests accurately reflect real-world performance.
- Test Early and Continuously: Incorporate performance testing with Jest into your development process early and continuously. Catching performance issues early in the development cycle can save time and effort in addressing them later. Continuously test and monitor performance throughout development to see any regressions or performance degradation.
- Use Realistic Workloads: Simulate realistic workloads in your performance tests. This includes simulating concurrent users, realistic request rates, and transaction volumes that reflect usage patterns. Avoid unrealistic or excessive workloads that may not accurately represent actual usage scenarios.
- Measure and Analyze Key Metrics: Define and measure key performance metrics, such as response time, throughput, error rates, and resource utilization. Analyze and interpret the performance metrics to identify performance bottlenecks and areas that need optimization. Use Jest’s built-in performance testing features, such as measuring test timings, to gather performance data.
- Optimize Test Execution: Optimize the execution of your performance tests to reduce interference from external factors. For example, isolate your performance tests from other resource-intensive tasks on the same machine, use headless browsers for UI performance testing, and disable unnecessary logging or debugging during performance tests.
- Monitor System Resources: Monitor system resources, such as CPU, memory, and network utilization, during performance testing. This can help identify resource-intensive operations and potential performance bottlenecks. Use monitoring tools like performance monitoring dashboards or system profiling tools to gain insights into system resource usage.
- Collaborate with Developers and Operations: Collaborate closely with developers and operations teams during performance testing. Involve them in the performance testing process, share performance results, and work together to identify and address performance issues. Collaboration between teams can lead to quicker resolution of performance issues and more effective performance optimizations.
- Document and Communicate Results: Document the performance testing process, results, and any optimizations. Communicate the findings to relevant stakeholders, including developers, operations, and business owners. Keep track of performance testing results over time to monitor performance trends and ensure that performance goals are consistently met.
Conclusion
Performance testing with Jest is a powerful tool for evaluating the speed and efficiency of your code. By utilizing Jest’s built-in performance testing capabilities, developers can identify bottlenecks, optimize performance, and ensure that their code meets the requirements of their users.
Developers can optimize their code for speed and efficiency through effective performance testing and improve their application’s overall user experience. It is important to note that performance testing is an ongoing process and should be conducted regularly throughout the development lifecycle.